AJAX stands for asynchronous javascript and xml. It was launched years ago but I found only one Blogger based blog with AJAX, beside that it is one of the coolest technology to adopt. Basically, when a visitor visits your blog, all the scripts (including CSS and Javascript) are loaded once, then whenever visits any page from the same site, a request is being sent to server, it returns data in plain text which would be much more lighter than the whole page and it will replace a space in DOM without refreshing the page. Maybe you are not familiar with it much, lets us dig into it.
A user action, it could be hover, click or any event but we are taking about clicking of link. When a user clicks a link or button, then a using Javascript or jQuery we send an AJAX request to server. Now the server returns data, the lighter the data would be, the faster it would be rendered. The rendered data is being displayed in HTML.
Now, during all this process not a single page reload takes place. If you are thinking is that possible in Blogger, then yes it is. From years we are fetching recent post using Javascript, that is also the same procedure, but it doesn't requires user action, the event was always set to pageLoad. While data was simple to handle, however creating the whole blog with AJAX is much more sensitive. Here is why?
How does it works?
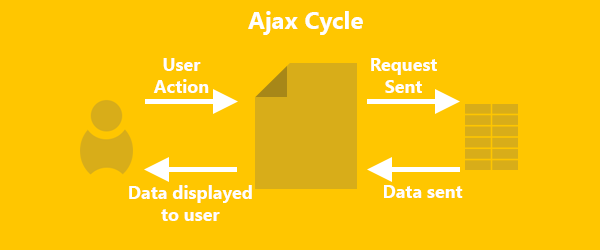
Now, during all this process not a single page reload takes place. If you are thinking is that possible in Blogger, then yes it is. From years we are fetching recent post using Javascript, that is also the same procedure, but it doesn't requires user action, the event was always set to pageLoad. While data was simple to handle, however creating the whole blog with AJAX is much more sensitive. Here is why?
Problems related to AJAX
There are problems with using AJAX, because this all involves Javascript which is being executed in browser. Unfortunately, search engine bots and social sites bots do not run Javascript. Not necessary user run it too. So here is the list all problems:
1. Javascript availability
The Javascript is required for AJAX request, almost every web surfer have their Javascript enabled. But some rare cases when user wants faster performance and would prefer to disable Javascript in browser. For such cases there solutions available, discussed later in the post.
2. SEO
Google and other search engine bots while indexing a site, never runs Javascript which obviously make the process shorter. AJAX content is loaded after the site loads, due to which nothing is left behind for a bot to index. However solutions are there to make it a search friendly technology.
3. Linking issues
A blog contains many internal links, either they could be in navigation or sidebar.Every internal link can be loaded via AJAX or simply by redirecting. If the link doesn't contain a standard markup then bots will never be able to index that link. Therefore careful handling of such cases are necessary.
4. Ads Impression
Ajax loaded websites faces great loss in adverts. Adsense and many others do not support AJAX for ads refreshing which is quite necessary to increase ads revenue, but since it is insecure for any advert agency therefore they do not prefer it.
5. Page views count
As far as I know Blogger's native page view stats doesn't work good with AJAX however Google Analytic's API have method to count each AJAX request on page even if page is not reloaded. To make sure that analysis is perfect, it is required to study Google Analytics' documentation carefully for such cases.
Introduction to Blogger V2
Blogger V2 is an updated and upgraded API which enable user to fetch data from Blogger's server using Javascript. Now what kind of resource we can get using this API.
- Blog:Displays all the posts including blog's meta data.
- Posts: Specific single post using Post Id
- Comments: Display comments by Post Id or single comment using comment Id.
- Pages: This each page using its Page Id.

Step 1 : Authentication
Since this all take place client side therefore it is required that we use some sort of key to make sure that no one else is accessing our blog's data. To create the key, go to Google Developer console. Then create a new project and click on credentials and request a new key. Now make sure you add domain of your blog so that every request from that specific domain may get the data.
Step 2 : Setup Makrup
Well markup matters the most. There are few things to note down:
- Page on load
- Internal links
- On internal link click
1. Page on load
In the beginning of the post I mentioned about bots indexing, if every page was there like it should be then there shouldn't be any problem. Therefore do not follow any of the below AJAX methods on a page on load while rendering. Means we will use those ordinary Blogger Layout data tags to display the content.2. Internal Links
Every links in post cannot be rendered with V2 API since post Id of each of them are not present. However, other all the links, that could be navigation, footer or async fetched post can have main link as async because we can get post or page id of each them. Further post describes it good.
3. On internal link click
Each post with post or page Id attribute can get into play with async stuff. Using the specific URL for each Id we can render the data quite easily and rapidly and that is the reason AJAX method works quite good in page loading speed.
How markup should look like?
A basic markup would contain a simple Blogger page (this is what I prefer due to SEO purpose). Here is the structure:
<header>
<!--Header-->
</header>
<div class="main-wrap">
<b:section class="main" id="main" showaddelement="no">
<b:widget id="Blog1" locked="false" title="Blog Posts" type="Blog">
...
<b:includable id="post" var="post">
</b:includable></b:widget></b:section><br />
<h2 class="post-title">
<a class="async-post" expr:data-postid="data:post.id" expr:href="data:post.url"><data:post.title/></a></h2>
...
</div>
<aside>
<!-- Sidebar -->
</aside>
In above code, the demonstration of normal Blogger template is placed. Inside var='post' we have the main post content. The link inside the main heading of post, a class, data-postid attribute and href attribute is added.What basically matters is that each link that can asynchronously fetched should have a specific class, in above code async-post class will make sure that the link should not redirect the page when clicked, however if Javascript would be disable then href attribute will work in normal conditions.
JSON data source
Below are listed of each type of resource we can use:
https://www.googleapis.com/blogger/v2/blogs/blogId/postsblogId, postId, pageId and comment Id must be replaced by unique id of each resource. Following data tags can be used to get these IDs.
https://www.googleapis.com/blogger/v2/blogs/blogId/posts/postId
https://www.googleapis.com/blogger/v2/blogs/blogId/posts/postId/comments
https://www.googleapis.com/blogger/v2/blogs/blogId/posts/postId/comments/commentId
https://www.googleapis.com/blogger/v2/blogs/blogId/pages
https://www.googleapis.com/blogger/v2/blogs/blogId/pages/pageId
Blog: <data:blog.id/>
Post: <data:post.id/>
Comment: <data:comment.id/>
Note: Post and Comments need to be executed in b:loop.
Final Javascript Code
The Javascript (jQuery) is used to send an AJAX request. Either you can use callback method which is conventional in blogs today or you can use an unconventional method of AJAX which I am going to use here, not because its easy but it also allows to select all links with ease instead of caring about loops and closures.
Just for example, the markup is below following:
<a href='http://google.com' data-postId='8398240586497962757' class='async-post'>Post</a>
<div></div>
The above code will create a link with href attribute and post id in data attribute. Then onclick of link, instead of refreshing the page, content with the below Javascript will be loaded in div.$(function() {
var link = $('.async-post'),
postId = link.attr('data-postId');
link.click(function() {
$.ajax({
type: 'GET',
url: 'https://www.googleapis.com/blogger/v2/blogs/Blog_Id_Here/posts/' + postId + '?key=YOUR_KEY',
async: false,
contentType: "application/json",
dataType: 'jsonp',
success: function(json) {
$('div').html(json.content)
}
});
return false;
});
});
I've just removed the key (for security sake). Just add your blog ID and key to make it work. It is just a demonstration and a demo of fetching a post.
Post A Comment:
0 comments: